/
Batch API Solution Concept
Batch API Solution Concept
Markus Geiss (Deactivated)
Rishabh Shukla (Unlicensed)
Owned by Markus Geiss (Deactivated)
The following document is in draft and subject to change.
Table of content
Summary
The Mifos X Batch API enables a consumer to access significant amounts of data in a single call or to make changes to several objects at once.
Batching allows a consumer to pass instructions for several operations in a single HTTP request. A consumer can also specify dependencies between related operations. Once all operations have been completed, a consolidated response will be passed back and the HTTP connection will be closed.
Solution
The Batch API takes in an array of logical HTTP requests represented as JSON arrays - each request has a requestId (the id of a request used to specify the sequence and as a dependency between requests), a method (corresponding to HTTP method GET/PUT/POST/DELETE etc.), a relativeUrl (the portion of the URL after https://example.org/api/v2/), optional headers array (corresponding to HTTP headers) and an optional body (for POST and PUT requests).
The Batch API returns an array of logical HTTP responses represented as JSON arrays - each response has a requestId, a status code, an optional headers array and an optional body (which is a JSON encoded string).
Definition
This is a sample request and sample response in JSON format. Here multiple requests are being sent to the API and multiple responses are being returned by it.
RequestBody Expand source
[ { "requestId" : 1, "relativeUrl" : "clients", "method" : "POST", "headers": [{"name": "Content-type", "value": "text/html"}, {"name": "X-Mifos-Platform-TenantId", "value": "text/html"}], "body" : "{ \"officeId\": 1, \"firstname\": \"Petra\", \"lastname\": \"Yton\", \"externalId\": \"76YYP7\", \"dateFormat\": \"dd MMMM yyyy\", \"locale\": \"en\", \"active\": true, \"activationDate\": \"04 March 2009\", \"submittedOnDate\": \"04 March 2009\", \"savingsProductId\" : 1 } " }, { "requestId" : 2, "relativeUrl" : "loans", "method" : "POST", "headers": [{"name": "Content-type", "value": "text/html"}], "reference": 1, "body" : "{ \"clientId\" : \"$.clientId\", \"productId\" : 1}" }, { "requestId" : 3, "method" : "POST", "relativeUrl" : "clients", "headers": [{"name": "Content-type", "value": "text/html"}], "body" : "{ \"officeId\": 1, \"fullname\": \"Pocahontas\", ... }" }, { "requestId" : 4, "method" : "POST", "relativeUrl" : "savingsaccount", "headers": [{"name": "Content-type", "value": "text/html"}], "reference": 3, "body" : "{ \"clientId\" : \"$.clientId\", \"productId\" : 1, ...}" } ]
ResponseBody Expand source
[ { "requestId" : 1, "statusCode" : 201, "headers" : [ { "name" : "Content-Type", "value" : "application/json; charset=UTF-8" } ], "body" : "{ \"officeId\": 1, \"clientId\": 1, ...}" }, { "requestId" : 2, "statusCode" : 201, "headers" : [ { "name" : "Content-Type", "value" : "application/json; charset=UTF-8" } ], "body" : "{ \"officeId\": 1, \"clientId\": 1, \"loanId\": 1, ...}" }, { "requestId" : 3, "statusCode" : 201, "headers" : [ { "name" : "Content-Type", "value" : "application/json; charset=UTF-8" } ], "body" : "{ \"officeId\": 1, \"clientId\": 2, ...}" }, { "requestId" : 4, "statusCode" : 201, "headers" : [ { "name" : "Content-Type", "value" : "application/json; charset=UTF-8" } ], "body" : "{ \"officeId\": 1, \"clientId\": 2, \"savingsId\": 1, ...}" } ]
Proposal
BatchApiResource acts like a proxy to BatchApiService and de-serializes incoming JSON to a set of BatchRequests and serialize a set of BatchResponses to JSON.
BatchApiService iterates the set of BatchRequests and obtaines the appropriate CommandStrategy from the CommandStrategyFactory. This utilizes the Strategy Pattern in combination with the Factory Pattern to ease the extension for future requirements.
The appropriate CommandStrategy executes the command using existing functionality and returns a BatchResponse.
Class Diagram
Sequence Digram
A sequence diagram for use case #1.
An agent has made a request to Mifos X API for the creation of a new client, along with other requests in the 'BatchRequest'. Different ApiResource classes are provided specific requests via BatchApiResource. In the final 'BatchResponse' all the responses from various ApiResource classes are concatenated together as a JSON array and this response is then sent back to the client.
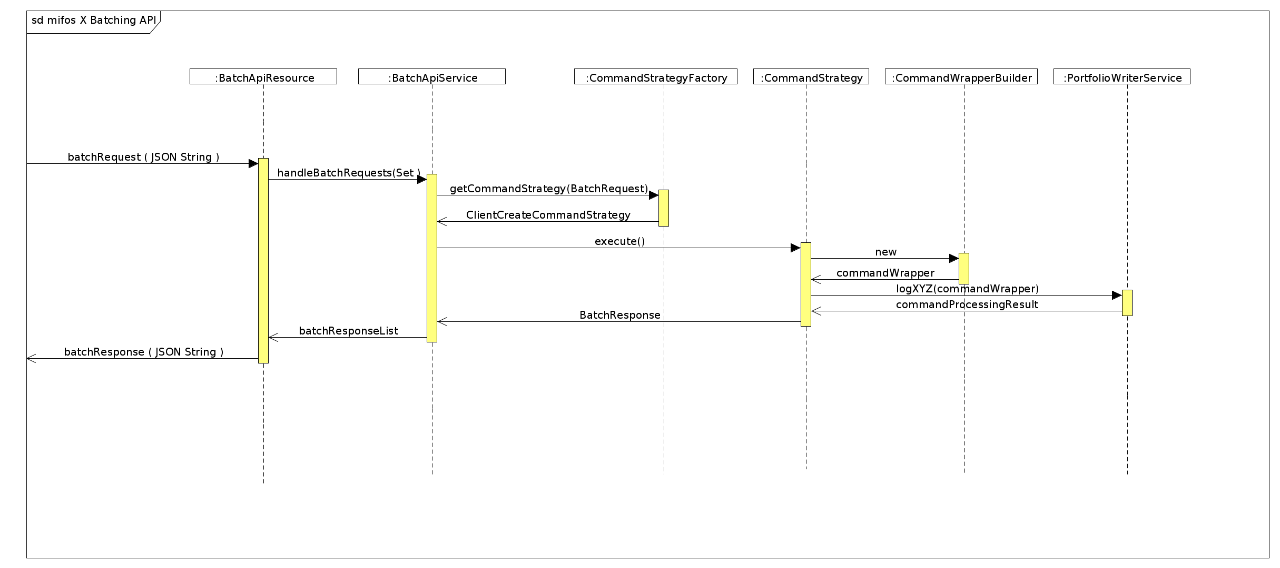
Milestones
- Create new packages and classes
- Add simple mock implementation for BatchApiService (including erroneous BatchResponses if requested)
- Implement use case 1 and 2
- Implement use case 3 and needed dependency resolution
- Implement use case 4 and needed dependency resolution
- Implement use case 5
- Extend community app and offer a collection sheet that uses the Batch API to retrieve and store multiple charges